Things you need to know about local storage in JavaScript

Ken Aqshal Bramasta / April 09, 2022
4 min read • ––– views
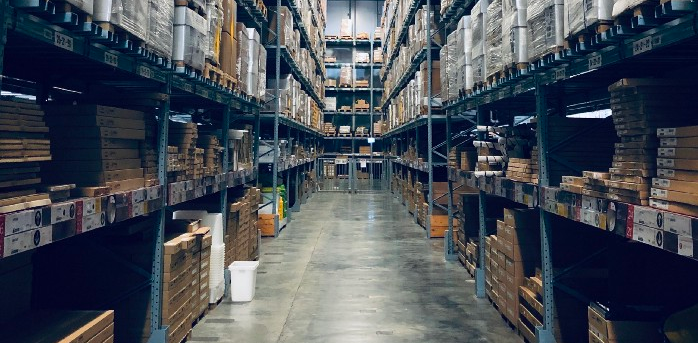
Table of Content
- What its local storage
- The Different between Local Storage and Session Storage
- Where is it being stored
- The function properties
- Things that you must note
What it's Local Storage
local Storage is a Storage mechanism that same as Session storage in Web Storage API, that allows you to save states like simple key-value store in object concept in JavaScript
The different mechanism between object and web storage stays intact during every page load. And the keys and the values are always stored in strings (note that, as with objects, integer keys will be automatically converted to strings)
The Different between Local Storage and Session Storage
the main concept that makes the two things different is the duration mechanism for storing the value. LocalStorage will never get expired even when the user closes and open the browser multiple time. However, if you use sessionStorage, the state will be clear when the browser is closed.
Where is it being stored?
LocalStorage is stored as an SQLite file under the user’s profile folder. if you use google chrome, it will be stored in \AppData\Local\Google\Chrome\User Data\Default\Local Storage
and in firefox, the browser will store it in webappsstore.sqlite
,
The Function Properties
key
The key()
method comes in handy in situations where you need to loop through keys and pass a number or index to retrieve the name of the key for the value that has already been set in Storage.
1var KeyName = localStorage.key(index);
setItem
this method allows you to store values in the storage
It takes two parameters: a key and a value itself. The key can be used later to fetch the value that you attached to it.
1localStorage.setItem('background', 'dark');
Where background
is the key and dark
is the value. Also, note that localStorage
can only store strings.
To store values that form in arrays and objects, you have to convert them to strings first before you can set them to the storage.
To do this, we use JSON.stringify()
method before we pass the value to setItem()
.
1//store object
2const animal = {name: "Crow", color: "Black"}
3localStorage.setItem('user', JSON.stringify(animal));
4
5//store array
6const arrAnimal = [{name: "Crow", color: "Black"}, {name: "Bull", color: "Red"}]
7localStorage.setItem('user', JSON.stringify(arrAnimal));
getItem
To get items from storage, we use the getItem()
method. the method allows you to access the data stored in the storage.
getItem()
accepts only one parameter, which is the key
that you use to set before, and returns the value
as a string.
To retrieve a user key:
1localStorage.getItem('animal');
This returns a string with the value as:
1"{name: 'Crow', color: 'Black'}"
Because the return value
always returns as string, you must convert it back to an object first.
To do that we can use JSON.parse()
method, so you can convert a JSON string into a JavaScript object.
1JSON.parse(localStorage.getItem('animal'));
removeItem
To delete local data, use the removeItem()
method.
When passed a key name in the removeItem()
method it will removes the key from storage if it exists. But if there is no item associated with the given key, the method will do nothing.
1localStorage.removeItem('animal');
clear
Use the clear()
method to reset all items that are already stored in storage.
when this method is invoked, it will clear the entire record in the storage in the domain, and It does not receive any parameters.
1localStorage.clear();
Things that you must note
Besides LocalStorage having a cool feature to help us store global value easily, it still has limits. below thing, you must note when you use local Storage is
- Do not use it to store sensitive user information in because
localStorage
is accessible to the user - Max size for storage data is limited to 5MB
- localstorage is not secure as it has no form of data protection and can be accessed by any code on your web page
localStorage
still adopts synchronous when the function is being execute, that means each operation must be run sequential
Enjoyed this post?
Check out some of my other articles: